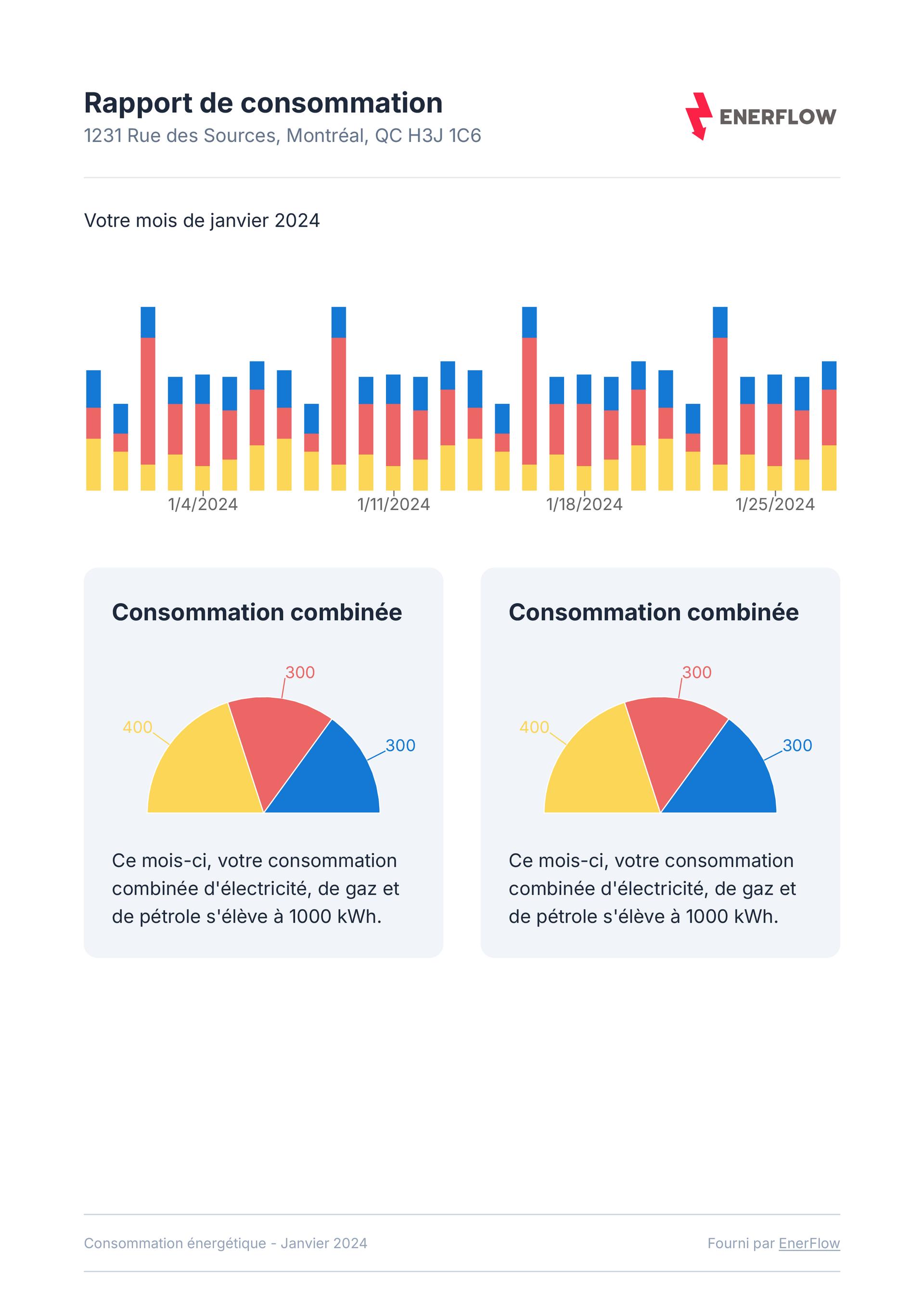
1 import { Footnote, PageBottom, Tailwind, CSS } from "@fileforge/react-print"; 2 import { 3 BarChart, 4 XAxis, 5 YAxis, 6 Bar, 7 Legend, 8 PieChart, 9 Pie, 10 Cell, 11 } from "recharts"; 12 13 export const dailyData = [ 14 { 15 date: new Date("2024-01-01").getTime(), 16 electricity: 4000, 17 gas: 2400, 18 oil: 2800, 19 }, 20 { 21 date: new Date("2024-01-02").getTime(), 22 electricity: 3000, 23 gas: 1398, 24 oil: 2210, 25 }, 26 { 27 date: new Date("2024-01-03").getTime(), 28 electricity: 2000, 29 gas: 9800, 30 oil: 2290, 31 }, 32 { 33 date: new Date("2024-01-04").getTime(), 34 electricity: 2780, 35 gas: 3908, 36 oil: 2000, 37 }, 38 { 39 date: new Date("2024-01-05").getTime(), 40 electricity: 1890, 41 gas: 4800, 42 oil: 2181, 43 }, 44 { 45 date: new Date("2024-01-06").getTime(), 46 electricity: 2390, 47 gas: 3800, 48 oil: 2500, 49 }, 50 { 51 date: new Date("2024-01-07").getTime(), 52 electricity: 3490, 53 gas: 4300, 54 oil: 2100, 55 }, 56 { 57 date: new Date("2024-01-08").getTime(), 58 electricity: 4000, 59 gas: 2400, 60 oil: 2800, 61 }, 62 { 63 date: new Date("2024-01-09").getTime(), 64 electricity: 3000, 65 gas: 1398, 66 oil: 2210, 67 }, 68 { 69 date: new Date("2024-01-10").getTime(), 70 electricity: 2000, 71 gas: 9800, 72 oil: 2290, 73 }, 74 { 75 date: new Date("2024-01-11").getTime(), 76 electricity: 2780, 77 gas: 3908, 78 oil: 2000, 79 }, 80 { 81 date: new Date("2024-01-12").getTime(), 82 electricity: 1890, 83 gas: 4800, 84 oil: 2181, 85 }, 86 { 87 date: new Date("2024-01-13").getTime(), 88 electricity: 2390, 89 gas: 3800, 90 oil: 2500, 91 }, 92 { 93 date: new Date("2024-01-14").getTime(), 94 electricity: 3490, 95 gas: 4300, 96 oil: 2100, 97 }, 98 { 99 date: new Date("2024-01-15").getTime(), 100 electricity: 4000, 101 gas: 2400, 102 oil: 2800, 103 }, 104 { 105 date: new Date("2024-01-16").getTime(), 106 electricity: 3000, 107 gas: 1398, 108 oil: 2210, 109 }, 110 { 111 date: new Date("2024-01-17").getTime(), 112 electricity: 2000, 113 gas: 9800, 114 oil: 2290, 115 }, 116 { 117 date: new Date("2024-01-18").getTime(), 118 electricity: 2780, 119 gas: 3908, 120 oil: 2000, 121 }, 122 { 123 date: new Date("2024-01-19").getTime(), 124 electricity: 1890, 125 gas: 4800, 126 oil: 2181, 127 }, 128 { 129 date: new Date("2024-01-20").getTime(), 130 electricity: 2390, 131 gas: 3800, 132 oil: 2500, 133 }, 134 { 135 date: new Date("2024-01-21").getTime(), 136 electricity: 3490, 137 gas: 4300, 138 oil: 2100, 139 }, 140 { 141 date: new Date("2024-01-22").getTime(), 142 electricity: 4000, 143 gas: 2400, 144 oil: 2800, 145 }, 146 { 147 date: new Date("2024-01-23").getTime(), 148 electricity: 3000, 149 gas: 1398, 150 oil: 2210, 151 }, 152 { 153 date: new Date("2024-01-24").getTime(), 154 electricity: 2000, 155 gas: 9800, 156 oil: 2290, 157 }, 158 { 159 date: new Date("2024-01-25").getTime(), 160 electricity: 2780, 161 gas: 3908, 162 oil: 2000, 163 }, 164 { 165 date: new Date("2024-01-26").getTime(), 166 electricity: 1890, 167 gas: 4800, 168 oil: 2181, 169 }, 170 { 171 date: new Date("2024-01-27").getTime(), 172 electricity: 2390, 173 gas: 3800, 174 oil: 2500, 175 }, 176 { 177 date: new Date("2024-01-28").getTime(), 178 electricity: 3490, 179 gas: 4300, 180 oil: 2100, 181 }, 182 ]; 183 184 export const synthesisData = [ 185 { 186 name: "Electricité", 187 value: 400, 188 }, 189 { 190 name: "Gaz", 191 value: 300, 192 }, 193 { 194 name: "Pétrole", 195 value: 300, 196 }, 197 ]; 198 199 <Tailwind> 200 <CSS>{`@import url('https://fonts.googleapis.com/css2?family=Inter:wght@400;700&display=swap'); 201 202 @page { 203 size: a4; 204 margin: .75in .75in 1in .75in; 205 } 206 `}</CSS> 207 208 <main className="text-slate-800 font-[inter]"> 209 <PageBottom> 210 <div className="text-xs text-slate-400 border-t border-t-slate-300 py-4 mt-4 flex border-b border-b-slate-300"> 211 <div>Consommation énergétique - Janvier 2024</div> 212 <div className="flex-grow" /> 213 <div> 214 Fourni par{" "} 215 <a 216 href="https://www.enerflow.ai/" 217 target="_blank" 218 className="underline underline-offset-2 " 219 > 220 EnerFlow 221 </a> 222 </div> 223 </div> 224 </PageBottom> 225 <div className="flex items-center pb-6 mb-6 border-b"> 226 <div className="flex-grow"> 227 <h1 className="font-bold text-2xl">Rapport de consommation</h1> 228 <p className="text-slate-500 "> 229 1231 Rue des Sources, Montréal, QC H3J 1C6 230 </p> 231 </div> 232 <img 233 src="https://framerusercontent.com/images/UM8IiaGXuEviaIXCXI1zvRJYd2k.svg" 234 className="h-12 block" 235 /> 236 </div> 237 <div className="my-6"> 238 <h2 className="mb-6">Votre mois de janvier 2024</h2> 239 <BarChart 240 width={730} 241 height={250} 242 data={dailyData} 243 margin={{ 244 top: 20, 245 left: 10, 246 right: 10, 247 }} 248 style={{ 249 width: "100%", 250 height: "auto", 251 }} 252 > 253 <XAxis 254 axisLine={false} 255 dataKey="date" 256 type="number" 257 scale="time" 258 domain={[ 259 new Date("2024-01-01").getTime(), 260 new Date("2024-01-14").getTime(), 261 ]} 262 tickFormatter={(date) => new Date(date).toLocaleDateString()} 263 ticks={dailyData 264 .map((d, i) => ((i + 3) % 7 === 0 ? d.date : null)) 265 .filter((d) => d !== null)} 266 /> 267 <Bar 268 barSize={13} 269 dataKey="electricity" 270 fill="#fcd656" 271 stroke="#fcd656" 272 stackId="1" 273 legendType="line" 274 name="Electricité" 275 /> 276 <Bar 277 barSize={13} 278 dataKey="gas" 279 fill="#ec6666" 280 stroke="#ec6666" 281 stackId="1" 282 legendType="line" 283 name="Gaz" 284 /> 285 <Bar 286 barSize={13} 287 dataKey="oil" 288 fill="#1479d4" 289 stroke="#1479d4" 290 stackId="1" 291 legendType="line" 292 name="Pétrole" 293 /> 294 </BarChart> 295 </div> 296 <div className="flex -mx-4"> 297 <div className="basis-0 flex-grow m-4 bg-slate-100 rounded-xl p-6"> 298 <h2 className="text-xl font-bold">Consommation combinée</h2> 299 <PieChart 300 width={300} 301 height={160} 302 className="my-6" 303 style={{ 304 width: "100%", 305 height: "auto", 306 }} 307 > 308 <Pie 309 cx="50%" 310 cy="100%" 311 outerRadius={115} 312 startAngle={180} 313 endAngle={0} 314 label 315 data={synthesisData} 316 dataKey="value" 317 > 318 {synthesisData.map((entry, index) => ( 319 <Cell 320 key={`cell-${index}`} 321 fill={ 322 index === 0 ? "#fcd656" : index === 1 ? "#ec6666" : "#1479d4" 323 } 324 /> 325 ))} 326 </Pie> 327 </PieChart> 328 <p> 329 Ce mois-ci, votre consommation combinée d'électricité, de gaz et de 330 pétrole s'élève à 1000 kWh. 331 </p> 332 </div> 333 <div className="basis-0 flex-grow m-4 bg-slate-100 rounded-xl p-6"> 334 <h2 className="text-xl font-bold">Consommation combinée</h2> 335 <PieChart 336 width={300} 337 height={160} 338 className="my-6" 339 style={{ 340 width: "100%", 341 height: "auto", 342 }} 343 > 344 <Pie 345 cx="50%" 346 cy="100%" 347 outerRadius={115} 348 startAngle={180} 349 endAngle={0} 350 label 351 data={synthesisData} 352 dataKey="value" 353 > 354 {synthesisData.map((entry, index) => ( 355 <Cell 356 key={`cell-${index}`} 357 fill={ 358 index === 0 ? "#fcd656" : index === 1 ? "#ec6666" : "#1479d4" 359 } 360 /> 361 ))} 362 </Pie> 363 </PieChart> 364 <p> 365 Ce mois-ci, votre consommation combinée d'électricité, de gaz et de 366 pétrole s'élève à 1000 kWh. 367 </p> 368 </div> 369 </div> 370 </main> 371 </Tailwind>;